Asynchronous vs. Synchronous Programming: Key Similarities and Differences
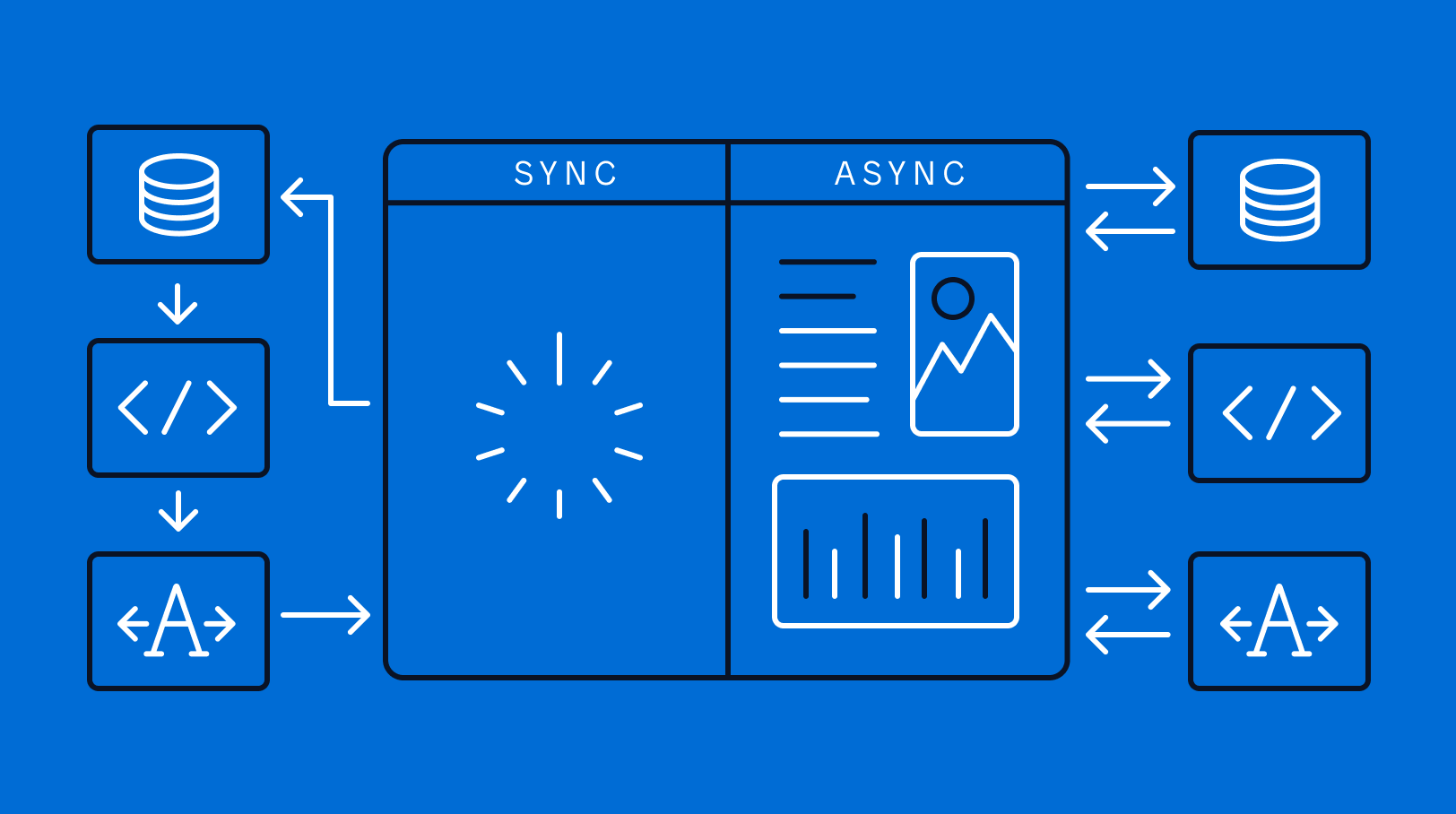
In computer programming and development, synchronous and asynchronous practices are essential—as long as you know the right model to use.
Synchronous tasks happen in order — you must finish the first job before moving on to the next. On the flip side, you can execute asynchronous jobs in any order or even simultaneously.
But how can this be understood in terms of programming?
Asynchronous and synchronous programming: What’s the difference?
Synchronous, sometimes called “sync,” and asynchronous, also known as “async,” are two different programming models.
Understanding how these two models differ is critical for:
- Building application programming interfaces (APIs)
- Creating event-based architectures
- Deciding how to handle long-running tasks
But before deciding which method to use and when, it’s important to know a few quick facts about synchronous and asynchronous programming.
Programming asynchronous applications
Asynchronous programming is a multithreaded model that’s applied to networking and communications.
Asynchronous is a non-blocking architecture, which means it doesn’t block further execution while one or more operations are in progress. With async programming, multiple related operations can run concurrently without waiting for other tasks to complete.
One way of programming asynchronous applications is with low-code application development. Multiple developers can work on projects simultaneously in a low-code platform, which helps accelerate the process of building apps.
Another example is texting. Texting is an asynchronous communication method. One person can text, and the recipient can respond at leisure. In the meantime, the sender may do other things while waiting for a response.
Programming synchronous applications
Synchronous is a blocking architecture and is best for programming reactive systems.
As a single-thread model, it follows a strict set of sequences, which means that operations are performed one at a time, in perfect order.
While one operation is being performed, other operations’ instructions are blocked. The completion of the first task triggers the next, and so on.
To illustrate how synchronous programming works, think of a telephone conversation. While one person speaks, the other listens. When the first person finishes, the second tends to respond immediately.
JavaScript
In the conversation about synchronous and asynchronous programming, there is a curveball called JavaScript.
JavaScript is a common scripting language that’s used to make websites interactive. JavaScript is an asynchronous and concurrent programming language that offers a lot of flexibility. It’s single-threaded like synchronous but also non-blocking like asynchronous.
Although it’s synchronous by nature, JavaScript benefits from an asynchronous process. Long-running JavaScript functions can make the user interface (UI) or server unresponsive until the function has returned, resulting in a less-than-stellar user experience.
However, there are some instances where users can benefit from blocking programming, for example, when making an online payment.
The benefit of JavaScript is that it offers the best of both worlds: Single-thread and multi-thread, blocking and non-blocking. With this flexibility, programmers can write code in a single programming language instead of two—one for synchronous operations and another for asynchronous operations.
Asynchronous vs. synchronous programming
Ultimately, the choice comes down to operational dependencies. Do you want the start of an operation to depend on another operation’s completion, or do you want it to run independently?
Asynchronous is a non-blocking architecture, so the execution of one task isn’t dependent on another. Tasks can run simultaneously.
Synchronous is a blocking architecture, so the execution of each operation depends on completing the one before it. Each task requires an answer before moving on to the next iteration.
The differences between asynchronous and synchronous include:
- Async is multi-thread, which means operations or programs can run in parallel.
- Sync is a single-thread, so only one operation or program will run at a time.
- Async is non-blocking, which means it will send multiple requests to a server.
- Sync is blocking — it will only send the server one request at a time and wait for that request to be answered by the server.
- Async increases throughput because multiple operations can run at the same time.
- Sync is slower and more methodical.
-
Asynchronous programming enhances the user experience by decreasing the lag time between when a function is called and when the value of that function is returned. Async programming translates to a faster, more seamless flow in the real world.
For example, users want their apps to run fast, but fetching data from an API takes time. In these cases, asynchronous programming helps the app screen load more quickly, improving the user experience.
Synchronous programming, on the other hand, is advantageous for developers. Synchronous programming is much easier to code. It’s well supported among all programming languages, and as the default programming method, developers don’t have to spend time learning something new that could open the door to bugs.
Async and sync use cases
Programming makes our digital world run, but without the right pairing of programs and operations, chaos and poor user experiences would ensue. Our digital world could spin into a mad, hyperactive frenzy if operations inappropriately rely on asynchronous programming.
And if operations are inappropriately relying on synchronous programming, our digital world could come to a screeching halt. It’s imperative to understand when to use each type of programming.
When to use async programming
Asynchronous programming is critical to programming independent tasks.
For instance, asynchronous programs are ideal for development projects with many iterations. Asynchronous programming will keep development moving forward because steps don’t have to follow a fixed sequence.
Responsive UI is a great use case for asynchronous planning. Take, for example, a shopping app. When a user pulls up their order, the font size should increase. Instead of first waiting to load the history and update the font size, asynchronous programming can make both actions happen simultaneously.
When to use sync programming
Asynchronous programming is relatively complex. It can overcomplicate things and make code difficult to read. On the other hand, synchronous programming is fairly straightforward; its code is easier to write and doesn’t require tracking and measuring process flows (as async does).
Because tasks depend on each other, you have to know if they can run independently without interrupting each other.
Synchronous programming could also be appropriate for a customer-facing shopping app. Users want to buy all their items together rather than individually when checking out online. Instead of completing an order every time the user adds something to their cart, synchronous programming ensures that the payment method and shipping destination for all items are selected at the same time.
How to choose between asynchronous and synchronous programming
When deciding which approach to take, consider asynchronous programming adaptable and synchronous programming strict.
Asynchronous programming is the multitasker, moving from one to-do to the other and alerting the system when each task is complete. Synchronous programming functions with a one-track mind, checking off one task at a time in a rigid sequence.
- Asynchronous programming allows more things to be done simultaneously and is typically used to enhance the user experience by providing an effortless, quick-loading flow.
- Synchronous programming is best utilized in reactive systems. While it is simpler for developers to code and is recognized by every programming language, sync is resource-intensive and can slow things down.
Frequently Asked Questions
-
Is JavaScript synchronous or asynchronous?
JavaScript is primarily synchronous, running one piece of code at a time. However, it can handle asynchronous tasks using callbacks, promises, and async/await. This allows JavaScript to remain responsive by doing other work while waiting for time-consuming operations to finish.
-
Is REST API synchronous or asynchronous?
REST APIs can be synchronous or asynchronous.
-
What are the three types of asynchronous programming?
The three types of asynchronous programming in JavaScript are callbacks, promises, and async/await:
-
Callbacks are functions passed as arguments to other functions to be executed later.
Promises represent a value that may be available now or in the future, allowing you to handle asynchronous operations more cleanly.
Async/await builds on promises, offering a more readable and straightforward way to write asynchronous code. -
Why is asynchronous programming better?
While asynchronous programming is not always the better option, it offers key benefits:
-
Improved responsiveness: Your app stays responsive during long tasks, preventing it from freezing.
Better efficiency: Multiple operations can run simultaneously, with results handled as they come in.
Enhanced user experience: Users can interact with other parts of your app without waiting for one task to finish. -
What is the drawback of asynchronous programming?
The main drawbacks of asynchronous programming are:
-
Increased code complexity: It can be harder to write and understand.
Harder to debug: Issues can be more challenging to track down.
More challenging error handling: Requires extra care to manage errors properly.
Synchronization issues: Multiple operations can conflict when accessing shared resources.